Many of us are looking into migrating their applications from the v2 stack over to v3. There are some good blog posts out there on the topic, but one thing that's sometimes overlooked are payload decoders/formatters.
From v2 to v3, their implementation has changed. Instead of 3 separate functions, there is now only one function doing all parts of the decoding, conversion and validation. Instead of having to rewrite these functions now, we've written a simple "adapter" function that allows you to use the v2 functions with the v3 stack.
You can copy all the TTN v2 converter functions into the editor field in the TTN v3 payload decoder configuration. Make sure to keep the original function names. If you didn't use one or more of the functions, you can just skip copy&pasting them. Then, add the following additional function at the bottom:
function decodeUplink(input) {
var data = input.bytes;
var valid = true;
if (typeof Decoder === "function") {
data = Decoder(data, input.fPort);
}
if (typeof Converter === "function") {
data = Converter(data, input.fPort);
}
if (typeof Validator === "function") {
valid = Validator(data, input.fPort);
}
if (valid) {
return {
data: data
};
} else {
return {
data: {},
errors: ["Invalid data received"]
};
}
}
With that, you don't need to do any further adaptations to your existing payload decoders, and can keep them split up into separate functions, which might be nice as well. I tested this with the Paxcounter, which only provides a Decoder function, and it works perfectly fine this way.
This was originally published in my blog, and you can find the code as a GitHub Gist as well.
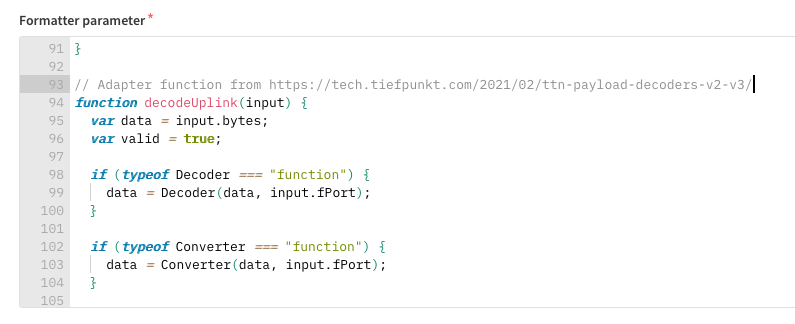